DOM Manipulation & Event Handling
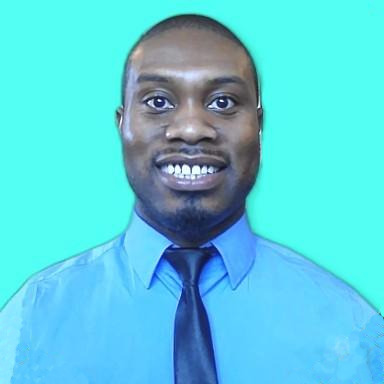
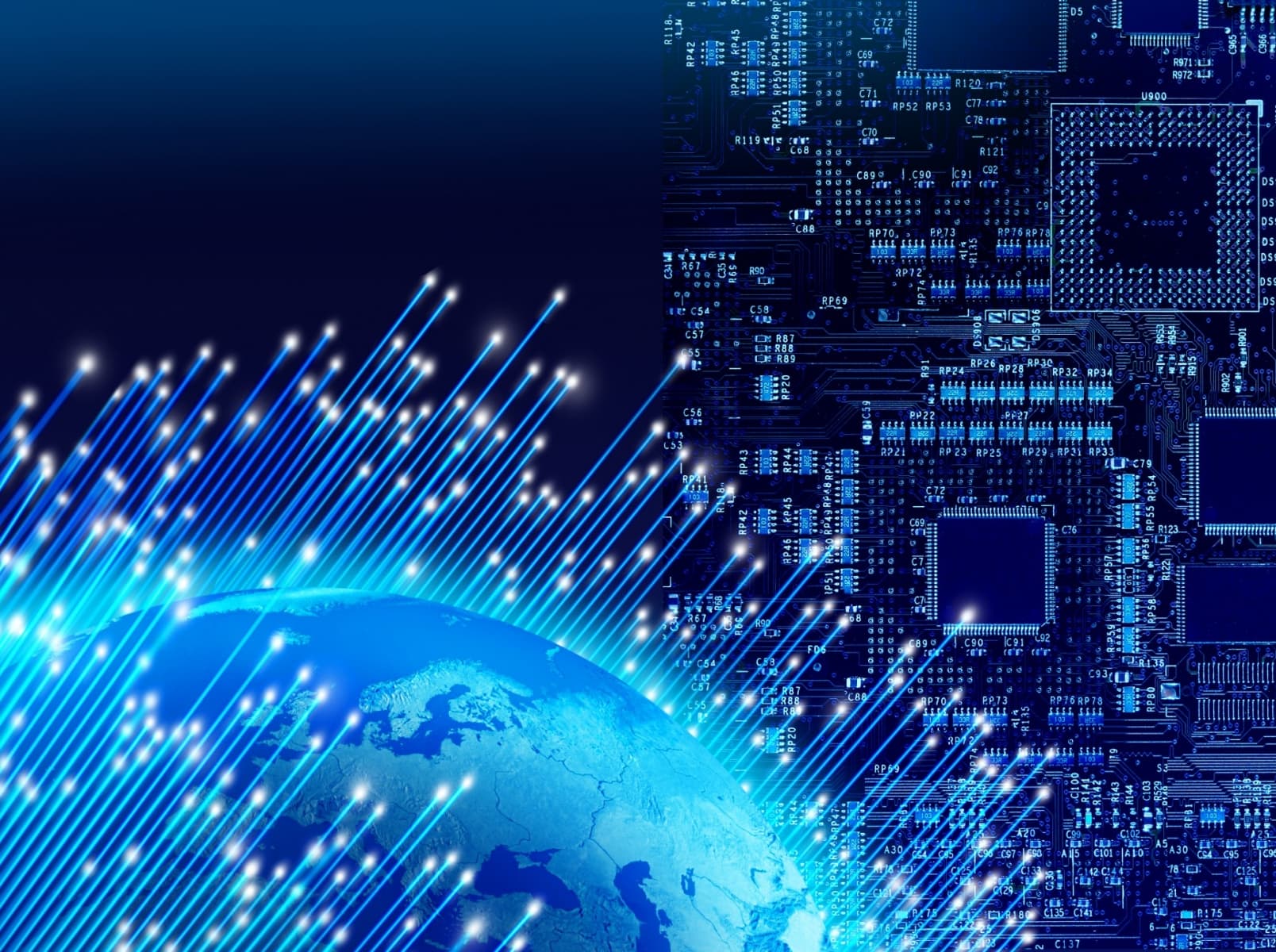
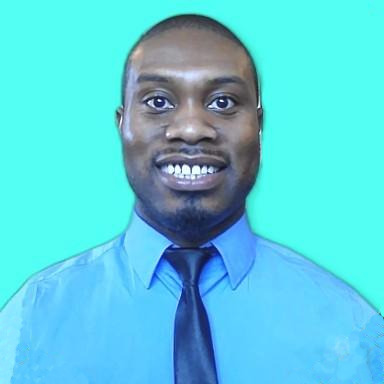
If you are someone who enjoys or is learning to enjoy manipulating the DOM, this article may be for you. It is not hard to see how the ability to create and edit the document structure of a browser using JavaScript in a unique way draws many developers in. These technical skills may or may not be key to your current or future workplace but honing them will only make you better. These skills are also acquired through education and practice so whether you choose to use them or not, increasing your knowledge base and recognition of other people’s code will be to your benefit.
Early stages
One good strategy is to determine if you are trying to create an element object from scratch or if you are trying to edit one that is already present. If you are creating something from nothing, the createElement() method is a great tool to utilize in your index.js file. If you are editing something already present on the DOM/your HTML file the document method querySelector() can be of great use to you. Along with the inspector tool you can search through the browser’s representation of your HTML file and see exactly how elements are organized and continue to work from there. If for some reason you prefer not to use the inspector tool you can make use of the DOM contains() method and check if a certain element is a descendant of another. This property will return a Boolean value revealing whether this is the case.
More methods
Another method that can make creating/editing in certain situations easier is the insertAdjacentHTML()
method which takes two arguments(position & text) in order to insert nodes at the desired location. The position argument must be one of the four strings pictured in the image below. The beforebegin
option will insert the element node before the element itself and the afterbegin
position option will place the node just inside the element, BEFORE its first child in the DOM Tree. The beforeend
choice will introduce the element just inside the element, AFTER its last child. Finally, the last available choice afterend
will position your targeted element after the element. Keep in mind that the beforebegin
and afterend
choices will only work if the HTML element is in the DOM tree and has a parent element. As you probably already ascertained, the second argument(‘text’) is the text content of your choosing. Thankfully, it also does not modify the existing elements already inside.
Class usage
Adding classes to elements is an effective way to group elements for easy identification and for future actions that you may or may not take. For example, by combining a callback function that allows you to iterate and a named NodeList, you can mass remove a group of elements from the DOM. It is important to be aware that one would be removing each element individually as you can only call remove()
on a singular element. The method querySelectorAll()
will enable you to group elements into a NodeList through use of CSS selectors.
Custom events
As events are just actions or occurrences and event handlers are just functions that are called whenever a specific event occurs, you could quickly create your own if you so choose. Although the available event reference lists on MDN, etc. are quite extensive, there may be times when a custom event may seem more suitable for a particular situation. In that case you can:
-
Call addEventListener on whichever element you are awaiting user interaction from and designate your responding function as the second argument.
-
Use and name new CustomEvent( ‘eventName’ ) to create the desired event. Use or do not use the detail property to pass additional custom data.
-
Then call
dispatchEvent
( ‘event’ ) on the DOM element(s) that you attachedaddEventListener
to trigger that event. -
Put your custom event’s name in as
addEventListener
's first argument.
Other tips
Using name attributes is a great way to access user input fields on a form and avoid errors. Be aware that the closest() method only works on the element itself and ancestor elements, not siblings. If you are editing something that is already there, be aware of how many divs/container tags are present on the page that you are working with to style your page in the manner that you intend. Lastly, keep up the good work.
Citations
“Creating and Triggering Events.” Event Reference | MDN, developer.mozilla.org/en-US/docs/Web/Events/Creating_and_triggering_events.